Your first web test
Prerequisites
> .Net 5 or greater installed (unicorn also works on .Net Framework >= 4.5.2, but steps will differ)
> Latest Unicorn.ConsoleRunner from
Unicorn tools downloads
(download and unpack it at any place on disk).
Create tests assembly
Create new library project. This will be your tests dll.
dotnet new classlib -o HelloWorldTests
cd HelloWorldTests
Install Unicorn packages for unit tests and Web UI + ChromeDriver package.
dotnet add package Unicorn.Taf.Core
dotnet add package Unicorn.UI.Core
dotnet add package Unicorn.UI.Web
dotnet add package Selenium.WebDriver.ChromeDriver
Add project property to copy all referenced assemblies into build directory into .csproj. Full scope of binaries is required to run tests.
<PropertyGroup>
<CopyLocalLockFileAssemblies>true</CopyLocalLockFileAssemblies>
</PropertyGroup>
Let's add config file for unicorn framework (let's name it unicornConfig.json). The file can contain configuration of unicorn framework.
In case of specific properties absence default values are used.
See Console runner page for config details.
In this demo the file will just contain some user defined properties (let's parameterize url to test website).
-- file should have CopyAlways or PreserveNewest attribute
-- file should not have UTF with BOM encoding
<ItemGroup>
<Content Include="unicornConfig.json">
<CopyToOutputDirectory>PreserveNewest</CopyToOutputDirectory>
</Content>
</ItemGroup>
File contents:
{
"userDefined": {
"siteUrl": "https://dobriyanchik.bitbucket.io/unicorn/test-ui-apps.html"
}
}
Application under test is 'Sample "Hello World" app' on Test UI apps page.
Let's check positive scenario that non-error modal window shows message for specified title and name.
Just add one more class which will contain test suite with a test.
using Unicorn.Taf.Core;
using Unicorn.Taf.Core.Testing;
using Unicorn.Taf.Core.Testing.Attributes;
using Unicorn.Taf.Core.Verification;
using Unicorn.Taf.Core.Verification.Matchers;
using Unicorn.UI.Core.Driver;
using Unicorn.UI.Core.Matchers;
using Unicorn.UI.Web;
using Unicorn.UI.Web.Controls;
using Unicorn.UI.Web.Controls.Typified;
using Unicorn.UI.Web.Driver;
namespace HelloWorldTests
{
// Test suite should be marked with [Suite] attribute and should inherit TestSuite.
[Suite("Sample tests")]
public class SampleTests : TestSuite
{
DesktopWebDriver driver;
// This code is executed before suite execution.
[BeforeSuite]
public void SuiteInit()
{
driver = new DesktopWebDriver(BrowserType.Chrome);
driver.Get(Config.GetUserDefinedSetting("siteUrl")); // Get test site url from unicorn config.
}
// Test should be marked with [Test] attribute.
[Test]
public void SimpleTest()
{
string title = "Mr";
string name = "NoName";
/* It's possible to search for not only generic WebControl, but also any custom or typified control.
* Main typified controls are from the box. All controls have built-in detailed logging.
* Available locators are xpath, css, id, name, class, tag. */
driver.Find<Dropdown>(ByLocator.Id("titleDropdown")).Select(title);
driver.Find<TextInput>(ByLocator.Id("name")).SetValue(name);
driver.Find<WebControl>(ByLocator.Css("a[href = '#helloWorld']")).Click();
WebControl modalWindow = driver.Find<WebControl>(ByLocator.Id("modalWindow"));
// Asserts from the box with rich matchers collection. Any matcher could be negated with Not matcher.
Assert.That(modalWindow, UI.Control.Visible());
string expectedMessage = $"{title} {name} said: 'Hello World!'";
// Chain asserts allow to make several checks without failing the test on first check fail.
new ChainAssert()
.That(modalWindow, Is.Not(UI.Control.HasAttributeContains("class", "error")))
.That(modalWindow, UI.Control.HasTextMatching($"\\s*{expectedMessage}\\s*"))
.AssertChain();
}
// This code is executed after suite execution.
[AfterSuite]
public void SuiteCleanUp() =>
driver.Close();
}
}
Run test
Build your tests assembly. Run next command within project directory:
dotnet build
Navigate to directory where Unicorn.ConsoleRunner was unpacked and execute the following command
Unicorn.ConsoleRunner.exe --test-assembly=path_to_HelloWorldTests.dll --config=path_to_unicornConfig.json
Congrats you have first passed unicorn test!
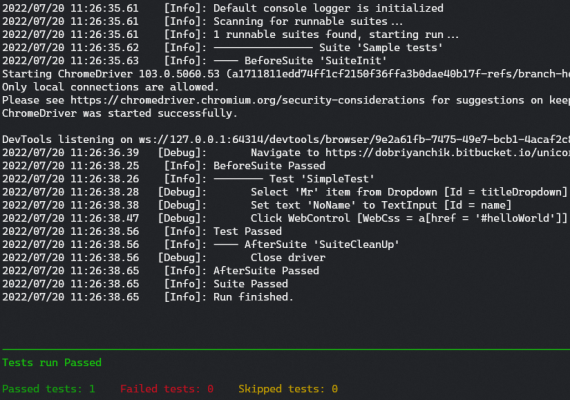